This project interfaces famous Microcontroller AT89C51 with LCD 16×2 with 3×4 Keypad. This project asks the user to enter password to unlock the lock or light up the LED.
Password security via keypad can be very productive you can just put a security lock outside your door and use a servo motor to unlock the door if the user enters a correct password. However, in this project we use a simple LED if the user enters a correct password LED will glow else not.
To see how to interface a simple keypad with LCD using AT89C51 please visit here.
LED will glow if the user enters a correct password i.e. 1234 in this case and to hide the original password not to display on the screen, LCD will show ‘x’ if a key is pressed. See the circuit diagram below:
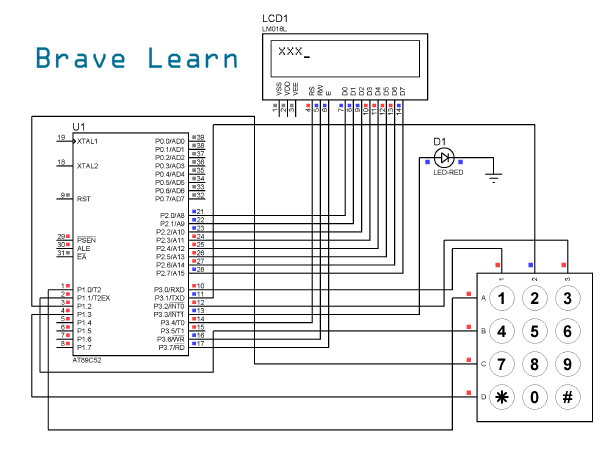
Now if a user enters a correct password i.e. 1234 in this case the LED will glow. However you may replace the LED with a relay and drive the servo motor which can be hooked up with your door lock etc. To know how to drive a relay with AT89C51 please visit here.
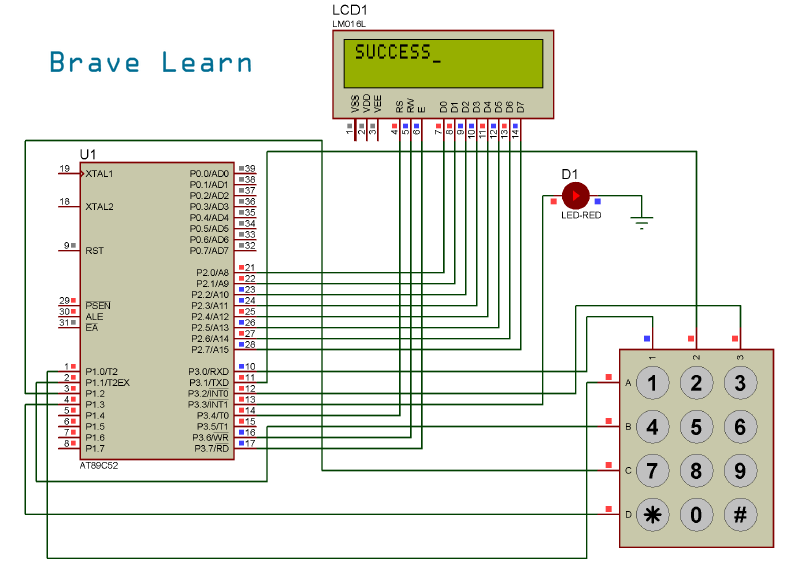
Now if a user enters the incorrect password the message “TRY AGAIN” will be displayed on the screen and LED will be turned OFF if it is already ON. You may enter the incorrect password to turn the lock ON i.e. switching the LED OFF after it is being turned ON.
You may modify the password as per you requirement in the code given below:
Code:
#include reg51.h
//LCD
sbit rs = P3^4; //register select pin
sbit rw = P3^6; //read write pin
sbit e = P3^7; //enable pin
sbit a = P1^0; //defines row A
sbit b = P1^1; //defines row B
sbit c = P1^2; //defines row C
sbit d = P1^3; //defines row D
sbit one = P3^0; //defines column one
sbit two = P3^1; //defines column two
sbit three = P3^2; //defines column three
sbit relay = P3^3;
int unlock;
void delay(unsigned int time) //Function to provide time delay in msec.
{
int i,j ;
for(i=0;i<time;i++)
for(j=0;j<1275;j++);
}
void lcdcmd(unsigned char item) //Function to send command to LCD
{
P2 = item;
rs= 0;
rw=0;
e=1;
delay(1);
e=0;
return;
}
void lcddata(double item) //Function to send data to LCD
{
P2 = item;
rs= 1;
rw=0;
e=1;
delay(1);
e=0;
return;
}
int read_num(){
//make all rows HIGH first
a = 1;
b = 1;
c = 1;
d = 1;
//check colomn by colomn to search for pressed key
one = 0; //Make column one LOW and search for keys press 1,4,7,*
two = 1; //Others remain HIGH
three = 1; //Others remain HIGH
if (a == 0) //If row A gets low means 1 is pressed
{return '1';}
if (b == 0) //If row B gets low means 4 is pressed
{return '4';}
if (c == 0) //If row C gets low means 7 is pressed
{return '7';}
if (d == 0) //If row D gets low means * is pressed
{return '*';}
//similar process for other keys
one = 1;
two = 0;
three = 1;
if (a == 0)
{return '2';}
if (b == 0)
{return '5';}
if (c == 0)
{return '8';}
if (d == 0)
{return '0';}
one = 1;
two = 1;
three = 0;
if (a == 0)
{return '3';}
if (b == 0)
{return '6';}
if (c == 0)
{return '9';}
if (d == 0)
{return '#';}
return -1; //if no button is pressed return -1
}
int check_pass(){ //SET Password 1234 and checks if the user enters the correct
int w,x,y,z;
w = read_num(); //reads password's first character
while (w == -1){
w = read_num();
}
lcddata('x'); //displays x to hide the data entered. This also confirms that the number has been entered
delay(100);
x = read_num(); //reads password's second character
while (x == -1){
x = read_num();
}
lcddata('x');
delay(100);
y = read_num(); //reads password's third character
while (y == -1){
y = read_num();
}
lcddata('x');
delay(100);
z = read_num(); //reads password's last character
while (z == -1){
z = read_num();
}
lcddata('x');
delay(100);
if (w=='1' && x == '2' && y == '3' && z == '4'){ //checks if the correct password entered i.e. 1234 you may also modify it to change the pass as u desire
return 1; //if password is correct return 1
}
else {
return 0; //if wrong pass is entered
}
}
void main()
{
relay = 0; //turn on the lock i.e. LED is Off
while(1){
lcdcmd(0x0E); //turn display ON for cursor blinking
lcdcmd(0x01); //clear screen
lcdcmd(0x06); //display ON
unlock = check_pass(); //asks to enter password and tells the variable unlock that it is correct or not
if (unlock == 1){ //unlock will be 1 if a correct pass 1234 is entered
lcdcmd(0x0E); //turn display ON for cursor blinking
lcdcmd(0x01); //clear screen
lcdcmd(0x06); //display ON
lcddata('S');
lcddata('U');
lcddata('C');
lcddata('C');
lcddata('E');
lcddata('S');
lcddata('S');
relay = 1;
delay (100);
}
else {
lcdcmd(0x0E); //turn display ON for cursor blinking
lcdcmd(0x01); //clear screen
lcdcmd(0x06); //display ON
lcddata('T');
lcddata('R');
lcddata('Y');
lcddata(' ');
lcddata('A');
lcddata('G');
lcddata('A');
lcddata('I');
lcddata('N');
delay(100);
relay = 0; //turn on the lock
}
}
}
Please burn the code using appropriate burner like G540 etc. To know how to burn code with G540 please visit here.
Have a Good Luck! running this simple project 🙂
If you have any suggestions or questions leave us a comment below: